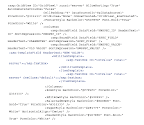
-------------------------------------------------------------
Just below it is the code with which you can access these individual textbox in the different rows.
-------------------------------------------------------------------------------------
for (int j = 0; j < GridView1.Rows.Count; j++)
{
Control cnt;
cnt = GridView1.Rows[j].Cells[3].Controls[1];
cnt = GridView1.Rows[j].FindControl("txtValue");
TextBox txtBx = (TextBox)cnt;
//write your code here to maniupulate the content of textbox
}